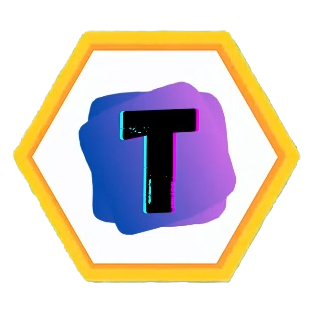
Texyland
Topic Checklist
- von Neumann architecture
- Basic C syntax, compiling programs
- Pointers and memory
- Static and global variables, variable scope
- Dynamic memory management in C (
malloc
and free
)
- Strings in C
- File handling in C (
FILE
, fopen
and fclose
)
- Sockets
- Concurrent programming
- Threads
- rwlock
- mutex
- spinlock
- Memory swapping, fragmentation and paging
- Process concept
- Process states
- Process Control Block (PCB)
- Resource sharing between "parent" and "child" processes
- Process termination (
wait
vs abort
)
- Context switching
- Scheduling processes
- Scheduling queues
- Scheduling criteria (measuring success)
- Scheduling algorithms (FCFS, SJF, PS, MLQS, processor affinity, load balancing)
- The critical-section problem
- Race conditions
- Synchronisation primitives
- Mutual exclusion
- Progress
- Bounded waiting
- Implementing a basic solution in C
- Semaphores
- File systems
- Interface exposed by the OS (logical view)
- How it's structured in memory (physical view)
- Caching
- Journaling file systems
- Disk access
- Steps involved to read/write
- Disk scheduling algorithms (FCFS, SSTF, SCAN, LOOK)
- Writing kernel programs in C
Please note: this is not an official University-released checklist, so use it as your own
discretion.